1. DHT11 Temp-humid Sensor¶
DHT11 is a temperature and humidity sensor.
1.1. Wiring¶
The DHT11 sensor in the lab is a three-pin sensor (shown below). Pins from left to right are Signal (green), Power (red) and Ground (black). Connect power pin to one of 3.3v pin on the Pi, signal pin to GPIO port, ground to GND.
In the example code, I connect the signal pin to BCM17 (physical pin 11)
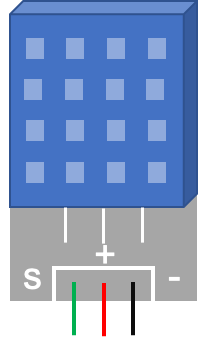
1.2. Programming¶
Download the library. Give credit to szazo DHT11_Python. You only need to download the
dht11.py
from the referred github or
Test code
#!/usr/bin/env python3
import RPi.GPIO as GPIO
from DHT11_lib import DHT11
import time
import datetime
# initialize GPIO
GPIO.setwarnings(False)
GPIO.setmode(GPIO.BCM)
GPIO.cleanup()
# read data using pin 14
instance = DHT11(pin=17) # BCM17 / Pin 11 change accordingly
while True:
result = instance.read()
if result.is_valid():
print("Last valid input: " + str(datetime.datetime.now()))
print("Temperature: %d C" % result.temperature)
print("Humidity: %d %%" % result.humidity)
time.sleep(1)
Expected output is:
Last valid input: 2019-04-06 20:45:04.533062
Temperature: 23 C
Humidity: 61 %
Last valid input: 2019-04-06 20:45:06.687475
Temperature: 24 C
Humidity: 30 %